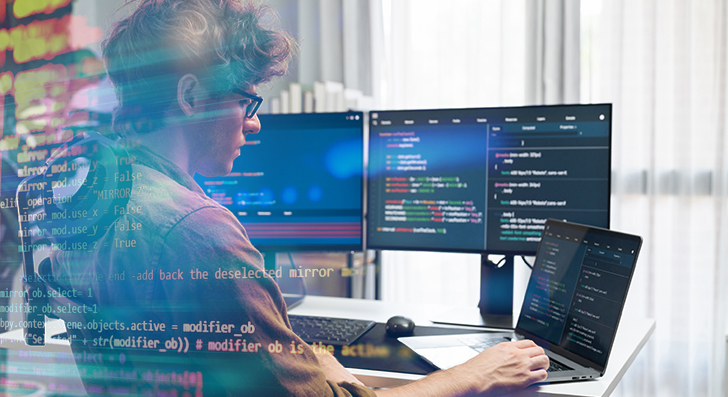
Scalability usually means your application can manage development—more people, far more details, and a lot more traffic—without breaking. To be a developer, constructing with scalability in mind will save time and tension afterwards. Listed here’s a transparent and practical guideline that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is just not anything you bolt on later—it should be element of your prepare from the beginning. A lot of purposes fall short every time they expand speedy due to the fact the original structure can’t manage the additional load. As a developer, you must think early about how your procedure will behave under pressure.
Begin by developing your architecture for being adaptable. Steer clear of monolithic codebases wherever every thing is tightly linked. In its place, use modular design and style or microservices. These patterns split your application into smaller, independent areas. Each module or support can scale By itself without impacting the whole system.
Also, contemplate your database from day one particular. Will it have to have to deal with 1,000,000 buyers or merely 100? Choose the correct sort—relational or NoSQL—dependant on how your data will develop. Program for sharding, indexing, and backups early, Even though you don’t have to have them but.
A different important level is to stop hardcoding assumptions. Don’t generate code that only is effective under present situations. Give thought to what would transpire In the event your user foundation doubled tomorrow. Would your app crash? Would the databases decelerate?
Use design styles that aid scaling, like information queues or function-driven techniques. These support your app manage far more requests with no acquiring overloaded.
Once you Construct with scalability in your mind, you are not just getting ready for success—you're decreasing future problems. A perfectly-prepared technique is less complicated to keep up, adapt, and expand. It’s superior to get ready early than to rebuild later.
Use the Right Databases
Picking out the proper database is a crucial A part of building scalable purposes. Not all databases are created the identical, and utilizing the Erroneous one can gradual you down as well as bring about failures as your app grows.
Start by knowledge your info. Can it be hugely structured, like rows inside a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great in shape. These are generally powerful with interactions, transactions, and consistency. In addition they assistance scaling procedures like go through replicas, indexing, and partitioning to take care of a lot more traffic and details.
When your data is much more flexible—like person activity logs, product or service catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at dealing with significant volumes of unstructured or semi-structured info and will scale horizontally much more quickly.
Also, think about your read through and generate patterns. Will you be doing many reads with fewer writes? Use caching and browse replicas. Are you presently handling a large produce load? Look into databases that will cope with high create throughput, as well as event-based mostly facts storage units like Apache Kafka (for temporary info streams).
It’s also sensible to Assume in advance. You may not require Innovative scaling features now, but choosing a database that supports them implies you gained’t have to have to modify later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your obtain styles. And always monitor database overall performance as you develop.
In brief, the proper database depends upon your app’s construction, pace requirements, And the way you anticipate it to develop. Consider time to pick sensibly—it’ll help you save loads of hassle afterwards.
Enhance Code and Queries
Speedy code is essential to scalability. As your app grows, each and every little delay provides up. Inadequately prepared code or unoptimized queries can slow down overall performance and overload your system. That’s why it’s imperative that you Develop efficient logic from the beginning.
Start off by composing clear, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most advanced Resolution if an easy a single works. Maintain your functions shorter, centered, and easy to check. Use profiling equipment to locate bottlenecks—sites in which your code will take as well very long to run or takes advantage of excessive memory.
Subsequent, evaluate your database queries. These normally sluggish matters down a lot more than the code itself. Be certain Every single question only asks for the information you truly want. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And stay away from executing too many joins, Primarily across massive tables.
If you detect exactly the same knowledge remaining requested over and over, use caching. Retail store the outcomes briefly utilizing equipment like Redis or Memcached this means you don’t need to repeat high-priced functions.
Also, batch your databases functions whenever you can. As an alternative to updating a row one after the other, update them in teams. This cuts down on overhead and makes your app a lot more efficient.
Remember to examination with massive datasets. Code and queries that do the job fine with 100 information may well crash if they have to take care of one million.
To put it briefly, scalable applications are fast apps. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's got to take care of more users and a lot more website traffic. If all the things goes as a result of a person server, it will eventually rapidly become a bottleneck. That’s where load balancing and caching are available. Both of these instruments enable maintain your app quick, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than 1 server doing all the do the job, the load balancer routes people to diverse servers determined by availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it can be reused immediately. When people request the same facts once again—like a product page or maybe a profile—you don’t must fetch it from the databases each time. You can provide it from the cache.
There's two typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static files near to the user.
Caching lowers databases load, enhances velocity, and tends to make your application much more successful.
Use caching for things that don’t improve typically. And always be sure your cache is current when info does improve.
In brief, load balancing and caching are uncomplicated but potent instruments. Together, they help your application tackle much more people, remain rapid, and recover from difficulties. If you intend to mature, you will need both equally.
Use Cloud and Container Applications
To construct scalable programs, you require tools that let your app increase quickly. That’s where cloud platforms and containers can be found in. They offer you adaptability, decrease setup time, and make scaling Significantly smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud System (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t really need to obtain components or guess upcoming potential. When traffic will increase, you may insert extra means with just some clicks or automatically using automobile-scaling. When site visitors drops, you'll be able to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You are able to concentrate on creating your app as an alternative to controlling infrastructure.
Containers are Yet another crucial Instrument. A container packages your application and anything it ought to operate—code, libraries, settings—into 1 device. This can make it uncomplicated to move your app concerning environments, from the laptop computer on the cloud, without having surprises. Docker is the most popular Software for this.
Once your app utilizes various containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If just one portion of one's application crashes, it restarts it mechanically.
Containers also ensure it is easy to different areas of your app into services. You may update or scale elements independently, which is perfect for overall performance and trustworthiness.
In a nutshell, using cloud and container instruments indicates you could scale quickly, deploy easily, and Get well quickly when troubles happen. In order for you your app to increase with no restrictions, commence applying these equipment early. They help you save time, minimize hazard, and enable you to keep centered on creating, not correcting.
Keep track of Almost everything
If you don’t check your software, you received’t know when issues go Mistaken. Checking assists the thing is how your app is executing, place difficulties early, and make better choices as your application grows. It’s a key A part of constructing scalable units.
Begin by tracking primary metrics like CPU use, memory, disk space, and response time. These inform you how your servers and expert services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this information.
Don’t just check your servers—keep an eye on your application much too. Regulate how long it takes for customers to load webpages, how often mistakes take place, and in which they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. By way of example, When your response read more time goes previously mentioned a Restrict or possibly a provider goes down, you ought to get notified right away. This aids you repair problems fast, normally right before people even observe.
Monitoring can also be useful after you make variations. In case you deploy a whole new function and see a spike in faults or slowdowns, it is possible to roll it back before it results in serious problems.
As your app grows, visitors and data raise. Without having monitoring, you’ll miss out on signs of trouble until eventually it’s also late. But with the right instruments in place, you continue to be in control.
To put it briefly, monitoring helps you maintain your app trusted and scalable. It’s not just about recognizing failures—it’s about knowing your procedure and making certain it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large providers. Even tiny applications need to have a strong foundation. By designing thoroughly, optimizing properly, and utilizing the proper applications, you'll be able to Establish apps that increase smoothly without having breaking stressed. Begin modest, Imagine huge, and Make intelligent.